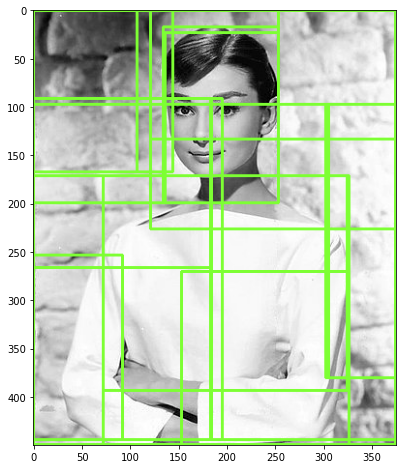
Published 2022. 4. 22. 23:44
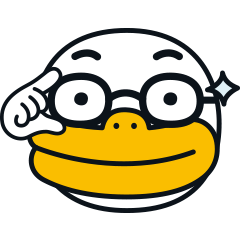
📌 이 글은 권철민님의 딥러닝 컴퓨터 비전 완벽 가이드 강의를 바탕으로 정리한 내용입니다.
목차
- selectivesearch 설치 및 이미지 로드
- 반환된 Region Proposal 정보 보기
- Bounding Box 시각화
selectivesearch 설치 및 이미지 로드
- 실습 환경: colab
- selective search 설치
!pip install selectivesearch
- 폴더 생성 및 이미지 로드
!mkdir /content/data
!wget -O /content/data/audrey01.jpg
- Python 모듈 추가 및 Rich output 표현을 위한 코드 작성
import selectivesearch
import cv2
import matplotlib.pyplot as plt
import os
%matplotlib inline
- cv2로 이미지 로드 및 시각화
img=cv2.imread('./data/audrey01.jpg')
img_rgb=cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
print('img shape:', img.shape)
plt.figure(figsize=(8,8))
plt.imshow(img_rgb)
plt.show()
반환된 Region Proposal 정보 보기
- 이미지의 Region Proposal 정보 가져오기
- selectivesearch.selective_search()
- 반환 자료형은 튜플
- 리스트 타입
- 세부 원소로 사전(dictionary)형 가지고 있다
- 개별 사전 내 Key값 별 의미
- rect 키 값은 x,y 시작 좌표와 너비, 높이 값을 가진다
- rect 키 값은 Detected Object 후보를 나타내는 Bounding Box
- rect의 첫번째와 세번째 항을 더해 가로 길이 계산
- rect의 두번째와 네번째 항을 더해 세로 길이 계산
- size는 Object의 크기
- labels는 해당 rect로 지정된 Bounding Box 내에 있는 Object들의 고유 ID
- 아래로 내려갈수록
- 너비와 높이 값이 큰 Bounding Box
- 하나의 Bounding Box에 여러 개의 Object가 있을 확률 증가
# 첫번째 변수명은 큰 의미가 없으므로 언더바로 표현
_, regions = selectivesearch.selective_search(img_rgb, scale=100, min_size=2000)
print(regions)
- rect 정보만 출력해서 보기
cand_rects = [cand['rect'] for cand in regions]
print(cand_rects)
Bounding Box 시각화
opencv의 rectangle()
- 인자 : 이미지와 좌상단 좌표, 우하단 좌표, box 컬러색, 두께
- 원본 이미지에 Bounding Box 그리는 함수
green_rgb = (125, 255, 51) # bounding box color
img_rgb_copy = img_rgb.copy() # 이미지 복사
for rect in cand_rects:
left = rect[0]
top = rect[1]
# rect[2], rect[3]은 너비와 높이이므로 우하단 좌표를 구하기 위해 좌상단 좌표에 각각을 더함.
right = left + rect[2]
bottom = top + rect[3]
img_rgb_copy = cv2.rectangle(img_rgb_copy, (left, top), (right, bottom), color=green_rgb, thickness=2)
plt.figure(figsize=(8, 8))
plt.imshow(img_rgb_copy)
plt.show()
- bounding box의 크기가 큰 후보만 추출
cand_rects = [cand['rect'] for cand in regions if cand['size']>10000]
# size가 10000보다 큰 경우만 추출
'🖼 Computer Vision > Object Detection' 카테고리의 다른 글
CV - Object Detection 주요 데이터셋 (0) | 2022.04.23 |
---|---|
CV - OD 성능 평가 Metric - mAP (0) | 2022.04.23 |
CV - NMS(Non Max Suppression) (0) | 2022.04.23 |
CV - OD 성능평가 Metric - IoU (Intersection over Union) (0) | 2022.04.23 |
CV - Object Detection의 이해 (0) | 2022.04.18 |