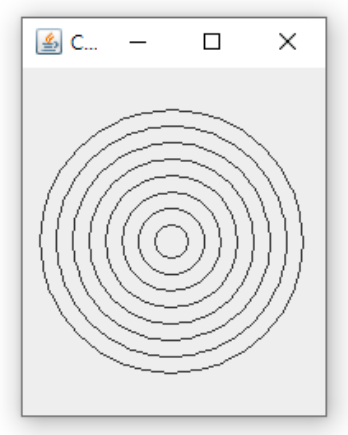
OOP Lab 14(4 x5=20%)
Due date : June 21, 23 : 59
Submit your assignment using the following file format: LabNumber_StudentName_Student_ID.zip
Example: Lab14_Hongkildong_201620505.zip.
Q1. The source code of an application that draws a series of eight concentric circles is given in the files “CirclesJPanel.java” and “Circles.java” in the folder “CodeQ1”. The circles are separated by 10 pixels. Hence, complete the partial source code in the file “Circles.java”. After your completed the code, the following figure should be displayed when you run the program.
· Requirement 1: the title for the frame is “Concentric Circles”.
· Requirement 2: the size for the frame is 200 x 250.
· Requirement 3: The number of circles are 8
· Requirement 4: The distance between two adjacent circles is 10 pixels.
// Circles.java
// This program draws concentric circles
import javax.swing.JFrame;
public class Circles extends JFrame
{
public static void main(String args[])
{
JFrame frame = new JFrame("Concentric Circles");
frame.setTitle("Concentric Circles");
frame.setSize(200, 250);
CirclesJPanel panel = new CirclesJPanel();
frame.add(panel);
frame.setVisible(true);
} // end main
} // end class Circles
// Requirement 1: the title for the frame is �Concentric Circles�.
// Requirement 2: the size for the frame is 200 x 250.
// CirclesJPanel.java
// This program draws concentric circles
import java.awt.Graphics;
import javax.swing.JPanel;
public class CirclesJPanel extends JPanel
{
// draw eight Circles separated by 10 pixels
public void paintComponent(Graphics g)
{
super.paintComponent(g);
// create 8 concentric circles
for (int topLeft = 0; topLeft < 80; topLeft += 10)
{
int radius = 160 - (topLeft * 2);
// read drawArc() method of Graphics class from java API
g.drawArc(topLeft + 10, topLeft + 25, radius, radius, 0, 360);
}
}
} // end class CirclesJPanel
Q2. Modify your solution to Q1 to draw the ovals by using Ellipse2D.Double class and draw () method of
Graphics2D class. Hence, complete the codes in files “Concentric.java” and “CirclesJPanel.java” under the folder CodeQ2. When you run the code after completing the code, the following figure is displayed.
// Concentric.java
// This program draws concentric circles using Graphics2D
import javax.swing.JFrame;
public class Concentric extends JFrame
{
public static void main(String args[])
{
JFrame frame = new JFrame("Concentric Circles");
frame.setTitle("Concentric Circles");
frame.setSize(250, 250);
CirclesJPanel2 panel = new CirclesJPanel2();
frame.add(panel);
frame.setVisible(true);
}
} // end class Concentric
// CirclesJPanel2.java
// This program draws concentric circles using Graphics2D
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.Ellipse2D;
import javax.swing.JPanel;
public class CirclesJPanel2 extends JPanel
{
// draw eight concentric circles separated by 10 pixels
public void paintComponent(Graphics g)
{
super.paintComponent(g);
// create Graphic 2D reference from g by filling the following Table
Graphics2D g2 = (Graphics2D) g;
for (int i = 0; i < 80; i += 10)
{
/*(30,30)is coordinate of upper left corner of
* enclosing rectangle of the outer circle
*/
int x= i+30;
int y= i+30;
/* find width and height upper left corner of
* upper left corner of enclosing rectangle of the outer circle
*/
int w= 160-(i*2);
int h= 160-(i*2);
// write your code to draw ellipse objects from Ellipse2D.Double class.
Ellipse2D.Double ellipse = new Ellipse2D.Double(x, y, w, h);
g2.draw(ellipse);
}
}
} // end class CirclesJPanel
// Hint 1: Read draw() of Graphics2D class from java API
// Hint 2: Read the constuctor Ellipse2D.Double(x,y,w,h) from java API
· Requirement 1: the title for the frame is “Concentric Circles”.
· Requirement 2: the size for the frame is 250 x 250.
· Requirement 3: The number of circles are 8
· Requirement 4: The distance between two adjacent circles is 10 pixels.
· Hint 1: refer the constructor of Ellipse2D.Double() s from java.awt.geom package ;
· Hint 2: refer the draw () method of Graphics2D class from java.awt.geom package
Q3. The source code of an application that draws ten nested rectangles using Rectangle2d.Double class is given. The rectangles are separated by 10 pixels on all sides. Complete the partial source code in the files “DrawRectangles.java” and “RectanglesPanel.java” under the folder CodeQ3. When you run the code, the following figure is displayed.
import javax.swing.JFrame;
public class DrawRectangles
{
public static void main(String arg[])
{
JFrame frame = new JFrame("Nested Rectangles");
frame.setTitle("Nested Rectangles");
frame.setSize(400, 400);
RectanglesPanel panel = new RectanglesPanel();
frame.add(panel);
frame.setVisible(true);
}
}
// Draw nested rectangles
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.Ellipse2D;
import java.awt.geom.Rectangle2D;
import javax.swing.JPanel;
public class RectanglesPanel extends JPanel
{
public void paintComponent(Graphics g)
{
super.paintComponent(g);
Graphics2D g2d=(Graphics2D)g;
int x=150, y=150; // coordinates of upper-left vertex of central rectangle
int width=50;
int length=30;// width and height of central rectangle
for(int i=1;i<=10;i++)
{
// write your code to draw ellipse objects from Ellipse2D.Double class.
g2d.drawRect(x, y, width, length);
x = x-10;
y = y-10;
width = width + 20;
length = length + 20;
}
}
}
· Requirement 1: the title for the frame is “Nested Rectangles”.
· Requirement 2: the size for the frame is 400 x 400.
· Requirement 3: The number of rectangles are 10
· Requirement 4: The distance between two adjacent rectangles is 10 pixel
Q4. The source code of an application that asks the user to input the radius of a circle as a floating-point number and then display the values of the circle’s diameter, circumference and area. Use the value 3.14159 for п. Complete the partial source code in the file “Circle.java” under CodeQ4. When you run the code, the following 4 figures are displayed sequentially.
· When you run the code, first, figure in (a) is displayed. · When you click “Ok” button after entering the value of radius, the figure in(b) is displayed, · When you click “Ok” button after entering the value of x-coordinate , the figure in(c) is displayed, · When you click “Ok” button after entering the value of y-coordinate, the figure in (d) is displayed.
|
// Circle.java
// Program calculates the area, circumference
// and diameter for a circle and draws the circle
import javax.swing.JFrame;
import javax.swing.JOptionPane;
public class Circle
{
public static void main(String args[])
{
JFrame frame = new JFrame();
String input1 = JOptionPane.showInputDialog(
null,"Enter radius:","input",JOptionPane.QUESTION_MESSAGE);
System.out.println(input1);
double inputRadius = Double.parseDouble(input1);
String input2 = JOptionPane.showInputDialog(
null,"Enter x-coordinate:","input",JOptionPane.QUESTION_MESSAGE);
int inputX = Integer.parseInt(input2);
String input3 = JOptionPane.showInputDialog(
null,"Enter y-coordinate:","input",JOptionPane.QUESTION_MESSAGE);
int inputY = Integer.parseInt(input3);
frame.setTitle("Circle");
frame.setSize(400, 400);
CirclesJPanel4 panel = new CirclesJPanel4(inputRadius, inputX, inputY);
frame.add(panel);
frame.setVisible(true);
}
} // end class Circle
프레임 먼저 만든다.
가장 첫 창인 input1을 만든다.
input1에서 입력된 값을 parseDouble로 받아서 inputRadius 에 저장해준다.
반복
// CirclesJPanel4.java
// Program calculates the area, circumference
// and diameter for a circle and draws the circle
import java.awt.geom.Ellipse2D;
import java.awt.Graphics;
import java.awt.Graphics2D;
import javax.swing.JPanel;
public class CirclesJPanel4 extends JPanel
{
private double radius;
private int x;
private int y;
// constructor initialize applet by obtaining values from user
public CirclesJPanel4(double inputRadius, int inputX, int inputY)
{
radius = inputRadius;
x = inputX;
y = inputY;
}
// draw results on applet's background
public void paintComponent(Graphics g)
{
Graphics2D g2d = (Graphics2D) g;
// to display string, do not use System.out.println();
g.drawString(String.format(
"Diameter is %f", (2 * radius)), 25, 30);
g.drawString(String.format(
"Area is %f", (Math.PI * radius * radius)), 25, 45);
g.drawString(String.format(
"Circumference is %f", (2 * Math.PI * radius)), 25, 60);
g2d.draw(new Ellipse2D.Double(x, y, radius, radius));
}
} // end class CirclesJPanel
a)
b)
c)
d)
참고 블로그
javacrush.tistory.com/entry/JOptionPane-입출력
[java] JOptionPane 사용자 입력창 사용 방법
JOptionPane 을 이용하면 사용자 입력창, 확인창, 알림창을 만들 수 있습니다. 기본적으로 매개변수는 동일하게 사용할 수 있으며 설명은 다음과 같습니다. 매개변수 설명: Object message 문자열 출�
javacrush.tistory.com
대화상자 (JOptionPane) 사용법
대화상자 (JOptionPane)이용하기. 대화상자(dialog box)를 사용하여 사용자에게 메시지를 보여주거나 간단한 데이터를 입력할 떄 입력대화상자를 사용할 수 있다 사용형식 JOptionPane.메소드이름(parentCom
iamkj.tistory.com
'📌 java > Object-oriented Programming' 카테고리의 다른 글
Homework_15 (0) | 2020.06.19 |
---|---|
Homework_13 (0) | 2020.06.09 |
java - ArrayList (0) | 2020.06.09 |
Homework_12 (0) | 2020.06.02 |
Homework_11 (0) | 2020.05.30 |