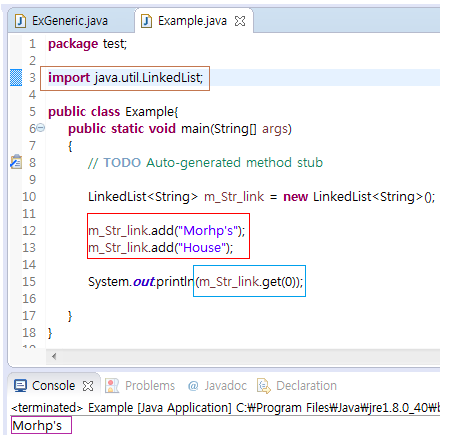
OOP Lab 13
Due Date: June 15, 23 : 59
· Submit your assignment using the following file format:
LabNumber_StudentName_Student_ID.zip
Example: Lab13_Hongkildong_201620505.zip
· The zip file will contain source code file that contains codes of classes.
Objectives
· How to use generic classes and interfaces that implements the basic data structures in computer Science
· Understand the advantage and disadvantage of each generic data structure classes
Exercises (7x5 =35%)
Q1.Modify lines 16-25 in Fig. 16.3 by using asList() method of Arrays class . This method converts static array colors and colors2 into generic data structure such as LinkedList. After converting color and color2 arrays, the LinkedList () constructor uses them as an argument to initialize list1 and list2 reference variables.
// Fig. 16.3 : ListTest.java
// Lists, LinkedLists and ListIterators
import java.util.List;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.ListIterator;
public class ListTest
{
public static void main(String[] args)
{
// add colors elements to list1
String[] colors =
{"black", "yellow", "green", "blue", "violet", "silver"};
List<String> list1 = new LinkedList<>(Arrays.asList(colors));
// add colors2 elements to list2
String[] colors2 =
{"gold", "white", "brown", "blue", "gray", "silver"};
List<String> list2 = new LinkedList<>(Arrays.asList(colors2));
list1.addAll(list2); //합집합
list2 = null; // release resources
printList(list1);
convertToUppercaseStrings(list1); // convert to upper case
printList(list1);
System.out.printf("%nDeleting elements 4 to 6 ...");
removeItems(list1, 4, 7); // re
printList(list1);
printReversedList(list1);
}
private static void removeItems(List<String> list, int start, int end)
{
list.subList(start, end).clear(); // remove items
}
private static void printReversedList(List<String> list)
{
ListIterator<String> iterator = list.listIterator(list.size());
System.out.printf("%nReversed List:%n");
// print list in reverse order
while (iterator.hasPrevious())
System.out.printf("%s ", iterator.previous());
}
// output List contents
private static void printList(List<String> list)
{
System.out.printf("%nlist:%n");
for (String color : list)
System.out.printf("%s ", color);
System.out.println();
}
// locate String objects and convert to uppercase
private static void convertToUppercaseStrings(List<String> list)
{
ListIterator<String> iterator = list.listIterator();
while (iterator.hasNext())
{
String color = iterator.next(); // get item
iterator.set(color.toUpperCase());
}
}
}
Q1. 어레이 클래스의 asList() 방법을 사용하여 그림 16.3의 16-25 라인을 수정하십시오.
이 메쏘드는 정적 배열 colors과 colors2를 LinkedList와 같은 generic 데이터 구조로 변환한다.
color와 color2 배열을 변환한 후 LinkedList() 생성자는 이를 인수로 사용하여 list1 및 list2 참조 변수를 초기화한다.
Arrays.asList()
Arrays 클래스의 asList() 메소드는 list 객체로 collection을 초기화하는데 편리한 방법을 제공해준다.
참고로 Java 8 기준으로 아직 java에는 set, map의 literal이 없다.
Q2. Write a program that reads in a series of first names and eliminates duplicates by storing them in a Set. Allow the user to search for a first name. The partial code is given in the file “ Q2Code”. Hence, complete the partial code by adding your own code.
Q2. 일련의 이름으로 읽고 중복된 것을 세트에 저장하여 제거하는 프로그램을 작성한다.
사용자가 이름을 검색할 수 있도록 허용해라.
// ListTest.java
// Program stores first names in Set.
import java.util.HashSet;
import java.util.Scanner;
import java.util.Set;
public class ListTest2
{
private static Scanner scanner = new Scanner(System.in);
public static void main(String args[])
{
Set<String> names = new HashSet<String>();
getNames(names); // get input from user
searchNames(names); // search for names
}
// get names
public static void getNames(Set<String> names)
{
// get name from standard input
System.out.println(
"Add a name to set, use end to terminate input:");
String inputName = scanner.next();
// obtain input until end entered
while (!inputName.equals("end"))
{
if(!names.contains(inputName)) //name did not exists in list
{
names.add(inputName);
System.out.println(inputName + " inserted");
}
else //name already exists in list
{
System.out.println(inputName + " exists in set");
}
System.out.println("Add a name to set, use end to terminate input:");
inputName = scanner.next();
}
}
// search names from list
private static void searchNames(Set<String> names)
{
// get name from standard input
System.out.println(
"Search a name, use end to terminate searching:");
String inputName = scanner.next();
// obtain input until end entered
while (!inputName.equals("end"))
{
// write code fill the blank space
boolean result = names.contains(inputName);
if(result) // name found
{
System.out.println(inputName + " found in set");
}
else // name not found
{
System.out.println(inputName + " not found in set");
}
// get next search name
System.out.println("Search a name, use end to terminate searching:");
inputName = scanner.next();
}
}
} // end class ListTest
Q3. Use a HashMap to create a reusable class for choosing one of the 13 pre-defined colors in a class Color. The names of the colors should be used as keys, and the predefined Color objects should be used as values. Place this class in a package that can be imported into any Java program. User your new class in an application that allows the user to select a color and draw a shape in that color. The partial code is given in the file “Q3Code”. Hence, complete the partial code by adding your own code.
// ColorChooser.java
// Class that uses a HashMap to store color-name and object pairs.
import java.awt.Color;
import java.util.HashMap;
import java.util.Set;
public class ColorChooser
{
private HashMap<String , Color> hashMap;
public ColorChooser()
{
hashMap = new HashMap<String, Color>();
// add the 13 colors to the hashMap
hashMap.put("black", Color.BLACK);
hashMap.put("blue", Color.BLUE);
hashMap.put("cyan", Color.CYAN);
hashMap.put("darkGray", Color.DARK_GRAY);
hashMap.put("gray", Color.GRAY);
hashMap.put("green", Color.GREEN);
hashMap.put("lightGray", Color.LIGHT_GRAY);
hashMap.put("magenta", Color.MAGENTA);
hashMap.put("orange", Color.ORANGE);
hashMap.put("pink", Color.PINK);
hashMap.put("red", Color.RED);
hashMap.put("white", Color.WHITE);
hashMap.put("yellow", Color.YELLOW);
}
// return the selected color
public Color getColor(String name)
{
return hashMap.get(name);
}
// return all the color names
public Set<String> getKeySet()
{
return hashMap.keySet();
}
} // end class ColorChooser
Q3. Color 클래스에서 미리 정의된 13가지 색상 중 하나를 선택하기 위해 재사용 가능한 클래스를 만들려면 해시맵을 사용하십시오.
색상의 이름은 키로 사용해야 하며, 미리 정의된 색 객체는 값으로 사용해야 한다. 이 클래스를 모든 Java 프로그램으로 가져올 수 있는 패키지에 넣으십시오. 사용자가 색상을 선택하고 해당 색상으로 모양을 그릴 수 있는 응용프로그램에서 새 클래스를 사용하십시오. 부분 코드는 "Q3Code" 파일에 주어진다. 따라서 자신의 코드를 추가하여 부분 코드를 완성하십시오.
Q4. Write an application to implement the three Set operations: interaction, union, and difference. You should build three methods corresponding to the operations. In your main method, test these methods on two HashSets of strings. The partial code is given in the file”Q4Code”. Hence, complete the partial code by adding your own code.
// SetOperations.java
import java.util.HashSet;
import java.util.Set;
public class SetOperations
{
public static Set<String> union(Set<String> s1, Set<String> s2)
{
HashSet<String> un=new HashSet<String>(s1);
un.addAll(s2);
return un;
}
public static Set<String> intersection(Set<String> s1, Set<String> s2)
{
Set<String> un=new HashSet<String>(s1);
un.retainAll(s2);
return un;
}
public static Set<String> difference(Set<String> s1, Set<String> s2)
{
Set<String> un=new HashSet<String>(s1);
un.removeAll(s2);
return un;
}
public static void main(String args[])
{
Set<String> footBallTeam=new HashSet<String>();
footBallTeam.add("Frank");
footBallTeam.add("Amjad");
footBallTeam.add("Jim");
Set<String> basketBallTeam=new HashSet<String>();
basketBallTeam.add("David");
basketBallTeam.add("Frank");
basketBallTeam.add("Scott");
Set<String> unionSet=union(footBallTeam,basketBallTeam);
Set<String> intersectionSet=intersection(footBallTeam,basketBallTeam);
Set<String> differenceSet=difference(footBallTeam,basketBallTeam);
System.out.println("unionSet :");
for(String member:unionSet)
System.out.println(member);
System.out.println("intersectionSet :");
for(String member:intersectionSet)
System.out.println(member);
System.out.println("differenceSet :");
for(String member:differenceSet)
System.out.println(member);
}
}// end of the class
Q4. 상호 작용, 결합, 차이의 세 가지 세트 운영을 구현하기 위한 신청서를 작성한다. 수술에 해당하는 세 가지 방법을 만들어야 한다. 기본 방법에서 이러한 방법을 두 개의 해시 집합 문자열에서 테스트하십시오. 부분 코드는 "Q4Code" 파일에 주어진다. 따라서 자신의 코드를 추가하여 부분 코드를 완성하십시오.
Q5. Write a program that has a method ListConcatenate() which receives two objects of LinkedList, and returns a new LinkedList that contains elements of the first list followed by elements of the second list. In the main method, test this method on two LinkedLists of strings.The partial code is given in the file “Q5Code”. Hence, complete the partial code by adding your own code.
import java.util.List;
import java.util.LinkedList;
public class ListConcatenation
{
public static LinkedList<String> listConcatenate(LinkedList<String> list1,LinkedList<String> list2)
{
LinkedList<String> list=new LinkedList<String>();
list.addAll(list1);
list.addAll(list2);
return list;
}
public static void main(String arg[])
{
LinkedList<String> list1=new LinkedList<String>();
list1.add("Java");
list1.add("C++");
LinkedList<String> list2=new LinkedList<String>();
list1.add("Pascal");
list1.add("Fortran");
LinkedList<String> list3=listConcatenate(list1,list2);
for (String s:list3)
System.out.println(s);
}
}
Q5. LinkedList의 두 개 객체를 수신하는 ListConcatenate() 메서드가 있는 프로그램을 작성하고, 첫 번째 목록의 요소와 두 번째 목록의 요소를 차례로 포함하는 새로운 LinkedList를 반환한다. 메인 메소드에서 문자열의 LinkedList 두 개에서 이 방법을 테스트하십시오. 부분 코드는 "Q5Code" 파일에 주어진다. 따라서 자신의 코드를 추가하여 부분 코드를 완성하십시오.
Q6. Write a program that uses a String method split to tokenize a line of text input by the user and places each token in a TreeSet. Print the elements of the TreeSet. [Note: This should cause the elements to be printed in ascending sorted order). The partial code is given in the file “Q6Code”. Hence, complete the partial code by adding your own code.
// TreeTest.java
// Program token sizes text input by user and places each
// token in a tree. Sorted tree elements are then printed.
import java.util.Scanner;
import java.util.TreeSet;
import java.util.StringTokenizer;
public class TreeTest
{
public static void main(String args[])
{
Scanner scanner = new Scanner(System.in); // create scanner
System.out.println("Please enter a line of text:");
String input = scanner.nextLine(); // get input text
TreeSet<String> tree = new TreeSet<String>();
StringTokenizer tokenizer = new StringTokenizer(input);
// add text to tree by filling the following blank spaces
/* Hint: StringTokenizer class has hasMoreTokens() and nextToken() methods.
you can refer these methods from Java API(java.util.StringTokenizer).
*/
while (tokenizer.hasMoreTokens())//
{ // token화 한 후 tree에 넣는다.
// add text to tree
tree.add(tokenizer.nextToken()); // nextToken : token 읽어오기
}
// print tree elements after adding each token
System.out.println("Elements in tree: " + tree);
}
} // end class TreeTest
Q6. String method split을 사용하여 사용자가 입력하는 텍스트 행을 토큰화하고 각 토큰을 TreeSet에 배치하는 프로그램을 작성한다. TreeSet의 요소를 인쇄하십시오. [주: 이렇게 하면 원소가 오름차순으로 인쇄될 수 있다. 부분 코드는 "Q6Code" 파일에 주어진다. 따라서 자신의 코드를 추가하여 부분 코드를 완성하십시오.
1. nextLine() 으로 입력
2. Token화 >> java.util.Stringtokenizer 이용
3. Token화 된 것을 TreeSet의 요소로 print
Q7. The output of Fig. 16.15 shows that PriorityQueue orders Double elements in ascending order. Rewrite Fig. 16.15 so that it orders Double elements in descending order (i.e., 9.8 should be the highest-priority element rather than 3.2). The partial code is given in the file “Q7Code”. Hence, complete the partial code by adding your own code.
// PriorityQueueTest.java
// Order PriorityQueue with a Comparator.
import java.util.Comparator;
import java.util.PriorityQueue;
public class PriorityQueueTest
{
public static void main(String[] args)
{
// queue of capacity 5 and a new Comparator
PriorityQueue<Double> queue =
new PriorityQueue<Double>(5, new DoubleComparator());
// insert elements to queue
queue.offer(3.2);
queue.offer(9.8);
queue.offer(5.4);
System.out.print("Polling from queue: ");
while (queue.size()> 0)
{
System.out.printf("%.1f ", queue.peek()); // 큐의 맨앞의 데이터를 뽑는다.
queue.poll(); // 큐의 맨 앞의 데이터를 추출한다.
}
}
private static class DoubleComparator implements Comparator<Double>
{
public int compare(Double first, Double second)
{
return second.compareTo(first);
}
}
} // end class PriorityQueueTest
Q7. 그림 16.15의 PriorityQueue의 출력은 오름차순으로 Double element가 표시된다.
그림 16.15를 내림차순으로 Double element를 출력하도록 다시 작성한다(즉, 9.8은 3.2가 아니라 가장 우선순위가 높은 요소가 되어야 한다). 부분 코드는 "Q7Code" 파일에 주어진다. 따라서 자신의 코드를 추가하여 부분 코드를 완성하십시오.
priorityQueue<> (5, new DoubleComparator());
에서 new DoubleComparator가 뭘 뜻하는 건지??? DoubleComparator 에 어떤걸 적어야하는건지 ?
위와 같은 질문이 있습니다.
LinkedList
"LinkedList" 클래스의 사용방법은 "ArrayList"의 사용방법과 동일하다.
왜냐하면 제공되는 메소드명이 동일하기 때문이다.
예를들어 삽입은 "add" 메소드이고, 삭제는 "remove" 메소드이다.
그럼 사용방법이 같은데 왜 따로 설명하느냐?
그건 내부에서 "ArrayList" 클래스와는 다르게 동작하는 것을 알아보기 위해서이다.
1. LinkedList 객체 생성/데이터 추가/데이터 사용
"LinkedList" 클래스의 객체 생성, 데이터 추가, 데이터 사용 방법은 "ArrayList" 클래스와 동일하다.
위 코드를 보자
우선 LinkedList 패키지를 import 해야한다.
그리고 객체 생성은 일반 클래스처럼 new 예약어로 생성한다.
그 후 데이터를 추가해 보자.
빨간 네모 부분을 보면 "add" 메소드로 추가해주는 모습이다.
또한 사용할 때는 "get"메소드로 인덱스를 넘겨서 사용하면 된다. 파란색 네모
이와 같은 내용은 "ArrayList" 클래스와 다 동일하다.
하지만 내부 구조는 "ArrayList" 클래스와 어떻게 다를까?
2. LinkedList 내부구조
"ArrayList" 클래스는 배열리스트로서 내부구조가 배열식으로 나열되어 있다.
반면에 "LinkedList" 클래스는 포인터로 링크되는 구조로 되어 있다.
위 그림을 보면 처음엔 "m_Str_link" 객체에는 아무것도 없다.
하지만 "add("Morph's")"를 한번 하니까 공간이 생겨서 데이터를 저장하게 된다.
여기까지는 ArrayList 클래스와 같지만 다음 데이터를 삽입할 때 구조가 달라진다.
"House" 문자열까지 "add"를 하고 나면 내부에서는 방이 각가 생성되고, 각 방은 서로 각각 링크를 걸어 가리키게 된다.
왜 연결하는 것일까?
ArrayList 클래스와 같이 배열 기반은 인덱스 번호가 있기 때문에 인덱스 번호로 해당 데이터의 위치를 찾을수가 있다.
하지만 LinkedList 클래스는 인덱스 번호 같은건 없다.
그래서 가장 첫번째 생성되는 방을 "root"로 하여 모든 데이터들을 저렇게 링크로 연결하게 된다.
여기서 데이터를 하나 추가하면 어떻게 될까?
이와 같이 "Welcome" 문자열을 추가하면 옆으로 길게 링크로 연결이 된다.
그러면 "get" 메소드로 데이터를 찾을 때 "root" 방으로부터 갯수를 세면서 데이터를 찾을 수가 있다.
"get(2)" 를 하게 되면 인덱스 2번을 바로 찾는게 아니고 "root" 부터 2번째까지 찾아가서 데이터를 가지고 오게 된다.
3. 중간 삽입 내부 구조
데이터와 데이터 사이에 값을 넣어줄 때는?
코드 사용법은 ArrayList와 동일하다.
내부적으로는 어떻게 될까?
1. 우선은 "Happy" 문자열의 공간을 만들고 문자를 넣어준다.
2. 그 다음 연결리스트 삽입하려고 하는 위치의 양옆에 있는 데이터와 링크를 걸어준다.
3. 그 다음 원래 연결되어 있던 링크를 끊어준다.
결론적으로 "Happy" 가 중간에 들어가는 꼴이 된다.
반드시 번호대로 해야한다.
4. 데이터 삭제
마지막으로 데이터 삭제이다.
"remove" 메소드를 활용하면 된다.
Morph's 와 Welcome 사이의 House를 삭제하려고 한다.
삭제되는 순서는 다음과 같다.
1. 삭제되려는 링크를 끊기 전에 양쪽에 있는 두 데이터를 서로 링크 걸어준다.
2. 그 다음 삭제할 데이터의 양쪽 링크를 끊는다.
'📌 java > Object-oriented Programming' 카테고리의 다른 글
Homework_15 (0) | 2020.06.19 |
---|---|
Homework_14 (0) | 2020.06.17 |
java - ArrayList (0) | 2020.06.09 |
Homework_12 (0) | 2020.06.02 |
Homework_11 (0) | 2020.05.30 |