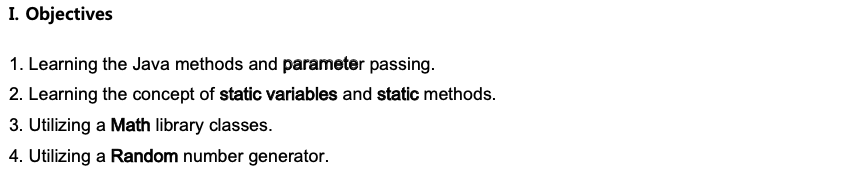
-
LabNumber _StudentName_StudentID.zip
(eg. Lab4_Hongkildong_201620505.zip
-
The zip file will contain two types of files, namely:
1) report file with file format “Report_Lab number” (eg. report_4) to write the answer of theory questions and the screen shots that display the output of your program.
2) Source code file that contains codes of classes to answer programming questions.
-
Submit your assignment using the following file format:
-
LabNumber _StudentName_StudentID.zip
(eg. Lab4_Hongkildong_201620505.zip
-
The zip file will contain two types of files, namely:
1. Java method 및 parameter 패스 학습.
2. 정적 변수와 정적 방법의 개념을 익힌다.
3. Math liabrary class 활용
4. 난수발생기 활용
ll. Questions (30 points)
1. Answer the questions about Fig 3.8 (Account.java).
A) At line 27, add transfer () method to the “Account” class as shown below.
Implement this method that transfer an “amt” from the one object of reference to another object reference of the “Account Class”.
If the money is transferred normally, the method return true. Otherwise, return false (3 points)
-> 27번 라인에서 아래와 같이 "Account" 클래스에 전송() 방법을 추가한다.
하나의 참조 개체에서 "Account class"의 다른 개체 참조로 "amt"를 전송하는 이 방법을 구현하십시오.
정상적으로 transfer되면 method은 true로 돌아온다.
그렇지 않으면 false(3점) ●
boolean transfer (Account b, double amt) {
// write your code here; }
B) Call the transfer () method after creating an object reference of “AccountTest Class” as shown Fig 3.9.
Compare the balance value before calling the transfer () method and after calling the transfer method() (including codes and captured screen) (3 points). ●
-> 그림 3.9와 같이 "AccountTestClass"의 객체 참조를 생성한 후 transfer() method을 호출하십시오.
2. Modify the Account class (Fig 3.8)
A) adding account number and initial account number as shown in the box below
아래 상자에 표시된 것처럼 계정 번호 및 초기 계정 번호 추가 ●
B) modifying the constructor as shown in the box below.
아래 박스에 표시된 바와 같이 생성자를 수정한다. ●
C) adding getInitilaAccountNumber() and getAccountAccountNumber() methods in the box below.
아래 상자에 getInitilaAccountNumber() 및 getAccountNumber() 메서드를 추가하십시오. ●
// Account number
private int accountNumber ;
// Variable to creating account numbers sequentially such as //1001, 1002, 1003, 104,105,106,...
private int initialAccountNumber = 1000;
// constructor
public Account(String name, double balance)
{
this.name = name;
if (balance > 0.0)
{
this.balance = balance;
}
// Initialize account number
acountNumber = getInitialAccountNumber();
}
// added method to create account number.
private int getInitialAccountNumber();
{
return ++ initialAccountNumber;
}
public int getAccountNumber ()
{
return accountNumber;
}
Question: In Fig 3.9, after creating three object reference variables of the “Account” class, print out the account numbers. However, you will not get correct result.
그림 3.9에서 "Account" 클래스의 객체 참조 변수 3개를 만든 후 계정 번호를 출력한다. 그러나, 당신은 정확한 결과를 얻지 못할 것이다.
A) Why the result is not correct (3 points).
accountNumber는 변할 수 있지만 initialAccountNumber는 초기값이므로 변하지 않아야 한다. 따라서 static 을 붙여야 한다.
B) Correct the code and capture the screen shot of your program (3 points)
3. In Fig.6.3, the class has two methods, namely main () and maximum ().
A) Modify this program by adding a third method called average () that computes the average of three numbers (Hint. You can add this method between line number 40 and 41) (3 points) ●
3개의 숫자의 평균을 계산하는 세 번째 method인 average()을 추가하여 이 프로그램을 수정한다. (힌트 : 40번과 41번 사이에 이 방법을 추가할 수 있음)(3점)
B) Modify also the body of main () method so that it will display the average value on the screen
(Hint: you can write between line 24 and line 25 ) (3 points) ●
main () 메서드의 본문도 수정하여 평균값을 화면에 표시하도록 한다.
(힌트: 24행과 25행 사이에 쓸 수 있음 )(3점)
4. Answer the following questions about Fig 6.3 (MaximumFinder.java).
A) Re-write the program by removing the “static” modifier of the maximum () method, and then compile the source code. Do you get the same result? Explain your reason. (3 points) ●
maximum() method의 "static" modifier를 제거하여 프로그램을 다시 작성한 후 source code를 컴파일해라. 같은 결과를 얻으십니까? 너의 이유를 설명해라. (3점)
Answer : 같은 결과를 얻지 못한다. Cannot make a static reference to the non-static method maximum()
B) Re-write the program by implementing the body of the maximum () method using the max () method of the Math class from the java.lang package (3 points). ●
Java.lang package에서 Math class의 max() max() method를 이용하여 max() method를 구현하여 프로그램을 다시 작성한다.
5. Answer the questions using the MethodOverload.java (Fig 6.10) codes.
A) Modify the code by adding the square(String stringValue) method as a form of method overloading as shown in the box below( Hint: add between line 27 and line 28)). This method returns a new string by concatenating the given string. Example, if the string “hello” is an argument, then it returns the string “hellohello”. After calling the square (String stringValue) method from main method ( Hint: between line 10 and line 11) and capture the screen shot of the program numbers. (3 points). ●
아래 박스와 같이 메서드 overloading의 형태로 square(String StringValue) 메서드를 추가하여 코드를 수정한다( 힌트: 27행과 28행 사이에 추가). 이 방법은 지정된 문자열을 연결하여 새 문자열을 반환한다.
예를 들어, "hello"라는 문자열이 인수인 경우, "hello" 문자열을 반환한다.
메인 메서드( 힌트: 10번과 11번 라인 사이)에서 스퀘어(String StringValue) 메서드를 호출한 후 프로그램 번호의 스크린샷을 캡처한다.(3점).
System.out.printf("Square of String 'hello' is %s%n", square("hello")); // call from main() method
public static String square(String stringValue) // header of the method
{
.....
}
B) Modify the program by adding the square (int n) method as method overloading as shown in the box below. This method takes an int value as an argument and return double type value as a square of the given integer value. The program will have an error. Why the program generates error. (3 points) ●
나) 아래 박스와 같이 overloading 메서드로 sqaure(int n) 방법을 추가하여 프로그램을 수정한다.
이 방법은 int 값을 인수로 사용하고 double형의 값을 주어진 정수 값의 제곱으로 반환한다.
프로그램에 오류가 있을 것이다. 프로그램에서 오류를 생성하는 이유(3점)
Answer : type of parameter value is 'integer', but type of return value is 'double'.
public static double square(int n) {
....
}
정적 변수와 메소드 (static)
이번에는 static에 대해서 알아보자. static은 "스태틱"이라고 읽는다.
static은 보통 변수나 메소드 앞에 static 키워드를 붙여서 사용하게 된다.
static 변수
예를 들어 다음과 같은 클래스가 있다고 하자.
public class HousePark {
String lastname = "박";
public static void main(String[] args) {
HousePark pey = new HousePark();
HousePark pes = new HousePark();
}
}
박씨 집안을 나타내는 HousePark이라는 클래스이다.
위와 같은 클래스를 만들고 객체를 생성하면 객체마다 객체변수 lastname을 저장하기 위한 메모리를 별도로 할당해야 한다.
하지만 가만히 생각해 보면 HousePark 클래스의 lastname은 어떤 객체이던지 동일한 값인 "박"이어야 할 것 같지 않은가?
이렇게 항상 값이 변하지 않는 경우라면 static 사용 시 메모리의 이점을 얻을 수 있다.
다음의 변경된 예제를 보자.
public class HousePark {
static String lastname = "박";
public static void main(String[] args) {
HousePark pey = new HousePark();
HousePark pes = new HousePark();
}
}
위와 같이 lastname 변수에 static 키워드를 붙이면 자바는 메모리 할당을 딱 한번만 하게 되어 메모리 사용에 이점을 볼 수 있게 된다.
※ 만약 HousePark 클래스의 lastname값이 변경되지 않기를 바란다면 static 키워드 앞에 final이라는 키워드를 붙이면 된다.
final 키워드는 한 번 설정되면 그 값을 변경하지 못하게 하는 기능이 있다. 변경하려고 하면 예외가 발생한다.
static을 사용하는 또 한가지 이유로 공유의 개념을 들 수 있다.
static 으로 설정하면 같은 곳의 메모리 주소만을 바라보기 때문에 static 변수의 값을 공유하게 되는 것이다.
다음의 예를 보면 더욱 명확하게 파악할 수 있을 것이다.
웹 사이트 방문시마다 조회수를 증가시키는 Counter 프로그램이 다음과 같이 있다고 가정 해 보자.
public class Counter {
int count = 0;
Counter() {
this.count++;
System.out.println(this.count);
}
public static void main(String[] args) {
Counter c1 = new Counter();
Counter c2 = new Counter();
}
}
프로그램을 수행해 보면 다음과 같은 결과값이 나온다.
c1, c2 객체 생성시 count 값을 1씩 증가하더라도
c1과 c2의 count는 서로 다른 메모리를 가리키고 있기 때문에
원하던 결과(카운트가 증가된)가 나오지 않는 것이다.
객체변수는 항상 독립적인 값을 갖기 때문에 당연한 결과이다.
이번에는 다음 예제를 보자.
public class Counter {
static int count = 0;
Counter() {
this.count++;
System.out.println(this.count);
}
public static void main(String[] args) {
Counter c1 = new Counter();
Counter c2 = new Counter();
}
}
int count = 0 앞에 static 키워드를 붙였더니 count 값이 공유되어 다음과 같이 방문자수가 증가된 결과값이 나오게 되었다.
보통 변수의 static 키워드는 프로그래밍 시 메모리의 효율보다는 두번째 처럼 공유하기 위한 용도로 훨씬 더 많이 사용하게 된다.
static method
static이라는 키워드가 메소드 앞에 붙으면 이 메소드는 스태틱 메소드(static method)가 된다.
무슨 말인지 알쏭달쏭하겠지만 예제를 보면 매우 쉽다.
public class Counter {
static int count = 0;
Counter() {
this.count++;
}
public static int getCount() {
return count;
}
public static void main(String[] args) {
Counter c1 = new Counter();
Counter c2 = new Counter();
System.out.println(Counter.getCount());
}
}
getCount() 라는 static 메소드가 추가되었다.
main 메소드에서 getCount() 메소드는 Counter.getCount() 와 같이 클래스를 통해 호출할 수 있게 된다.
※ static 메소드 안에서는 인스턴스 변수 접근이 불가능 하다.
위 예에서 count는 static 변수이기 때문에 스태틱 메소드(static method)에서 접근이 가능한 것이다.
보통 스태틱 메소드는 유틸리티성 메소드를 작성할 때 많이 사용된다.
예를 들어 "오늘의 날짜 구하기", "숫자에 콤마 추가하기"등의 메소드를 작성할 때에는 클래스 메소드를 사용하는 것이 유리하다.
다음은 "날짜"를 구하는 Util 클래스의 예이다.
import java.text.SimpleDateFormat;
import java.util.Date;
public class Util {
public static String getCurrentDate(String fmt) {
SimpleDateFormat sdf = new SimpleDateFormat(fmt);
return sdf.format(new Date());
}
public static void main(String[] args) {
System.out.println(Util.getCurrentDate("yyyyMMdd"));
}
}
Util클래스의 getCurrentDate라는 스태틱 메소드(static method)를 이용하여 오늘의 날짜를(yyyyMMdd) 구하는 예제이다.
위 클래스를 실행하면 오늘의 날짜가 출력될 것이다.
자바를 한번쯤 공부해본사람이라면 static키워드를 모르지는 않을 것입니다.
하지만, 바르게 알고 있는 사람들은 그리 많지 않습니다.
자바경력자를 면접볼 때 static키워드에 대해서 질문하곤 합니다.
면접관 : static키워드에 대해서 설명해보세요.
응시자 : static키워드를 쓰면, 객체를 생성하지 않고도 변수나 함수를 사용할 수 있습니다.
면접관 : 왜 static키워드를 쓰나요?
응시자 : 객체를 생성하지 않아도 되니까 편리하고 속도도 빠릅니다.
면접관 : 그렇다면 모든 변수와 함수에 static을 붙이는 것이 좋겠네요?
응시자 : 가능한한 static을 붙이는 것이 좋다고 생각합니다.
면접관 : 어떤 경우에 static을 붙일 수 있고, 어떤 경우에 static을 붙일 수 없습니까?
응시자 : ...
면접관 : 만일 당신이 새로운 클래스를 작성한다고 할 때, 어떤 경우에 static키워드를
사용해야한다고 생각합니까?
응시자 : ...
대부분의 경우 위와 같은 내용으로 문답이 진행됩니다.
사실 응시자의 대답은 다 맞는 얘기입니다.
하지만, static의 핵심적인 개념을 모르기 때문에 어떤 경우에 왜 static을 사용해야하는지는 잘모르는 것 같습니다.
먼저 결론부터 간단히 정리하면 다음과 같습니다.
1. 클래스를 설계할 때, 멤버변수 중 모든 인스턴스에 공통적으로 사용해야하는 것에 static을 붙인다.
- 인스턴스를 생성하면, 각 인스턴스들은 서로 독립적이기 때문에 서로 다른 값을 유지한다. 경우에 따라서는 각 인스턴스들이 공통적으로 같은 값이 유지되어야 하는 경우 static을 붙인다.
2. static이 붙은 멤버변수는 인스턴스를 생성하지 않아도 사용할 수 있다.
- static이 붙은 멤버변수(클래스변수)는 클래스가 메모리에 올라갈때 이미 자동적으로 생성되기 때문이다.
3. static이 붙은 메서드(함수)에서는 인스턴스 변수를 사용할 수 없다.
- static이 붙은 메서드는 인스턴스 생성 없이 호출 가능한 반면, 인스턴스변수는 인스턴스를 생성해야만 존재하기 때문에...
static이 붙은 메서드(클래스메서드)를 호출할 때 인스턴스가 생성되어 있을 수도, 그렇지 않을 수도 있어서
static이 붙은 메서드에서 인스턴스 변수의 사용을 허용하지 않는다.
(반대로, 인스턴스 변수(static 안 붙은 메서드)나 인스턴스 메서드(static 안 붙은 메서드)에서는 static이 붙은 멤버들을 사용하는 것이 언제나 가능하다. 인스턴스 변수가 존재한다는 것은 static이 붙은 변수가 이미 메모리에 존재한다는 것을 의미하기 때문이다.)
4. 메서드 내에서 인스턴스 변수를 사용하지 않는다면, static을 붙이는 것을 고려한다.
- 메서드의 작업 내용 중에서 인스턴스 변수를 필요로 한다면, static을 붙일 수 없다. 반대로 인스턴스변수를 필요로 하지 않는다면, 가능하면 static을 붙이는 것이 좋다. 메서드 호출시간이 짧아지기 때문에 효율이 높아진다. (static을 안 붙인 메서드는 실행시 호출 되어야 할 메서드를 찾는 과정이 추가적으로 필요하기 때문에 시간이 더 걸린다.)
5. 클래스 설계시 static의 사용지침
- 먼저 클래스의 멤버변수중 모든 인스턴스에 공통된 값을 유지해야하는 것이 있는지
살펴보고 있으면, static을 붙여준다.
- 작성한 메서드 중에서 인스턴스 변수를 사용하지 않는 메서드에 대해서 static을
붙일 것을 고려한다.
일반적으로 인스턴스변수와 관련된 작업을 하는 메서드는 인스턴스메서드(static이 안붙은 메서드)이고 static변수(클래스변수)와 관련된 작업을 하는 메서드는 클래스메서드static이 붙은 메서드)라고 보면 된다.
다음은 static에 대한 자세한 설명과 예제입니다.
static은 객체지향개념을 이해하는 가장 중요한 첫걸음이니 확실히 알아두셔야합니다.
==================================================================
'📌 java > Object-oriented Programming' 카테고리의 다른 글
Homework_W6 (0) | 2020.04.23 |
---|---|
Homework_W5 (0) | 2020.04.14 |
Homework_W3 (0) | 2020.03.31 |
java - accesor, mutator (0) | 2020.03.31 |
java - method call (0) | 2020.03.31 |