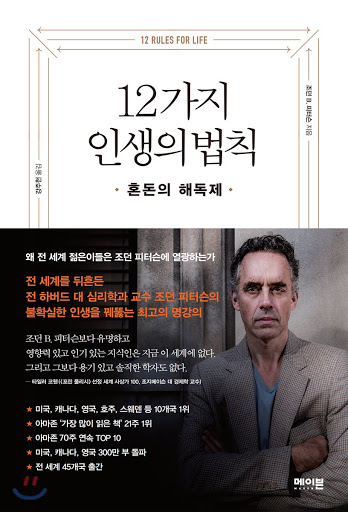
OOP Lab3: Due Date: April 05, 2020
· Submit your assignment using the following file format:
Week_Number_StudentID_ Name.zip (eg. W3_201721057 _Hongkildong.zip).
· This zip file will contain source code file to answer programming questions.
I. Objectives (total score: 15)
III. Programming Problems
1. Problem Description
We want to develop a program which manages the purchasing of products. There are 10 different types of products in a stock. Each product has a unique identification number from 1 to 10. We want to maintain the price of each product type, the identification number of each product type and the number of items of each kind of product that are purchased.
우리는 제품 구매를 관리하는 프로그램을 개발하고 싶다.
재고품에는 10가지 종류의 상품이 있다.
각 제품은 1부터 10까지의 고유 식별 번호를 가지고 있다.
제품 종류별 가격, 제품종류별 식별번호, 구매품목 수를 유지하고자 한다.
A customer can buy several products at once. For example, you can show purchasing information such as:
· 1 item from product type 5,
· 4 items from product 2,
· 7 items from product type 1.
고객은 한 번에 여러 제품을 살 수 있다. 예를 들어 다음과 같은 구매 정보를 표시할 수 있다.●
· 제품 타입 5에서 1개 항목
· 제품 타입 2에서 4개 항목
· 제품 타입 1에서 7개 항목
Given the above information of a product, the program should perform the following functions.
1. View the unit price of a product
2. Add an item to the information system when a product is purchased
3. Calculate the total price of a product
제품에 대한 위의 정보를 고려할 때 프로그램은 다음 기능을 수행해야 한다.
1. 제품 단가 보기 ●
2. 제품 구매 시 정보 시스템에 항목 추가 ●
3. 제품의 총가격을 산정한다 ●
1. Detailed Description of each function
a) View the unit price of a product: Scan the product identification number and show the price of the product( 5pt)
제품 단가 보기: 제품 식별 번호 검색 후 제품 가격 표시(5pt) ●
b) Add a purchasing product to the system(5pt):
Scan the product identification number and save the price of the item of the purchased product.
If this product is already in a purchasing list, add the quantity (number) of the product.
Subtract the number (quantity) of the purchased product in stock.
If the quantity of a selected product in the stock is not sufficient (the total stock of the product is less than the number of products to be purchased), display the error message “the product is out of stock” on the screen.
시스템에 구매 제품 추가(5pt):
제품 식별 번호를 스캔하여 구매한 제품의 가격을 저장하십시오. ●
이 제품이 이미 구매 목록에 있는 경우 제품의 수량(번호)을 추가하십시오. ●
재고로 구매한 제품의 수량(수량)을 뺀다. ●
재고에서 선택한 상품의 수량이 충분하지 않은 경우(제품의 총 재고량이 구매할 제품 수보다 적음), "제품 품절"이라는 오류 메시지를 화면에 표시한다. ●
C) Calculate the total price (5pt): Display the total price of the purchased items and exit the program.
총 가격 계산(5pt): 구매한 상품의 총 가격을 표시하고 프로그램을 종료한다. ●
2. User Interface Requirements
· Once the program starts, the program should display to the user the menu to select one of the above three function.
· “View the unit price” and “Add a purchasing product” function can be performed repeatedly.
· Finally selecting “Calculate the total price” function and terminate the program
· 프로그램이 시작되면, 프로그램은 위의 세 가지 기능 중 하나를 선택할 수 있는 메뉴를 사용자에게 표시해야 한다.
· "단가보기" 및 "구매상품 추가" 기능을 반복적으로 실시할 수 있다. ●
· 최종적으로 "총 가격 산정" 기능 선택 및 프로그램 종료 ●
int[] unit_price;
void view_unit_price(...) { ... }
· Initial value of product price information or stock information can be used and configured in program.
· How to use basic array in java ?
· 제품가격정보나 제고정보의 초기값 프로그램에 활용, 구성할 수 있다.
· 자바에서 기본배열을 사용하는 방법?●
a) int[] unit_price = {10, 20, 30, 40, 50, 60, 70, 80, 90, 100};
// when initialize integer array, assign the size.
b) int[] unit_price = new int[10]; // create array to save 10 integer dynamically
Question: Write the entire program and attach the screen shot of the running program.
My code
import java.util.Scanner;
public class Store {
public static void main (String[] args) {
Scanner select = new Scanner(System.in);
int[][] unit = {{1, 10, 0},
{2, 20, 25},
{3, 30, 25},
{4, 40, 25},
{5, 50, 25},
{6, 60, 25},
{7, 70, 25},
{8, 80, 25},
{9, 90, 25},
{10, 100, 25},
};
int menu;
int total_price = 0;
while (true)
{
System.out.println("\n1. View the unit price\n"
+ "2. Add a purchasing product\n"
+ "3. Calculate the total price\n"
+ "Select the number. What do you like to do?");
menu = select.nextInt();
if (menu == 1)
{
System.out.println("\nWhat kind of products would you like to see?\n"
+ "Please enter the number");
int n = select.nextInt();
if (n <= 10) {
System.out.println("product type:" + unit[n-1][0] +
"\nproduct price:" + unit[n-1][1] +
"\nproduct stock:" + unit[n-1][2]);
}
else
{
System.out.println("We don't have the product");
}
}
else if (menu == 2)
{
//message
System.out.println("What type?"); // type
int i = select.nextInt();
if (i <= 10)
{
System.out.println("How many?"); // count
int j = select.nextInt();
if(unit[i-1][2] - j >= 0)
{
total_price += unit[i-1][1]*j;
unit[i-1][2] = unit[i-1][2] - j; // 제고 빼주기
}
else //(unit[i][3] - j < 0)
{
System.out.println("The product is out of stock");
}
}
else
{
System.out.println("We don't have the product.");
}
}
else if (menu == 3) // (menu == 3)
{
System.out.println("Total price is " + total_price);//total price
break;
}
else
{
System.out.println("You choose wrong number.\n");
}
}
}
}
배열 정의하기
package org.opentutorials.javatutorials.array;
public class GetDemo {
public static void main(String[] args) {
String[] classGroup = { "최진혁", "최유빈", "한이람", "이고잉" };
System.out.println(classGroup[0]);
System.out.println(classGroup[1]);
System.out.println(classGroup[2]);
System.out.println(classGroup[3]);
}
}
int[] unit_price = {10, 20, 30, 40, 50, 60, 70, 80, 90, 100};
int[] unit_price = new int[10]; // 배열 만들건데 10개를 만들거다
unit_price[0] = 10;
System.out.println(unit_price.length); // 변수의 개수를 출력한다. 10이 출력된다.
'📌 java > Object-oriented Programming' 카테고리의 다른 글
Homework_W5 (0) | 2020.04.14 |
---|---|
Homework_W4 (0) | 2020.04.07 |
java - accesor, mutator (0) | 2020.03.31 |
java - method call (0) | 2020.03.31 |
java - public의 의미 (0) | 2020.03.27 |