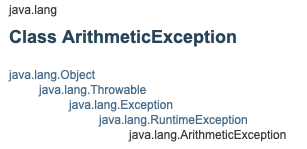
OOP Lab 10
Due Date: May 22, 23 : 59
I. Objectives
1. Learning the structure of Exception Handling.
2. The five key words in Exception handling structure: try, catch, finally, throws and throw keywords
3. Learning the difference between Checked Exceptions and Unchecked Exceptions.
II. Questions(20 points)
1. Answer the following questions after executing the code in Fig11.3 (refer the slide)
A. At which line number the “InputMismatchException” occur? (1 point)
Answer :
25 line number : int numerator = scanner.nextInt();
27 line number : int denominator = scanner.nextInt();
B. Why the “InputMismatchException” occurs? (1 point)
Answer :
Because data type of "numerator" and "denominator are integer.
So we must input the integer type.
C. At which line number the “ArithmeticException” occurs? (1 point)
Answer : 29 line number : int result = quotient(numerator, denominator);
D. Why the “ArithmeticException” occurs. (1 point)
Answer : If we input the integer '0', integer cannot divde by zero.
2. Answer the following questions about the program in Fig 11.3 (refer the slide)
A. What happens if you add and compile the following catch block between line 33 and line 34 in Fig. 11.3? Please including only your explanation(2 points)
Answer : It cannot compiled.
That's because the catch statement that appears after RuntimeException is a non-executable phrase because RuntimeException means a more comprehensive exception than InputMismatchException, ArthematicException.
Java compilers detect unnecessary logic and inform developers of it.
그것은 RuntimeException이 InputMismatchException, ArithemeticException 보다 포괄적인 예외를 의미하기 때문에 RuntimeException 이후에 등장하는 catch 문은 실행될 수 없는 구문이기 때문이다. 자바 컴파일러가 불필요한 로직을 감지하고 이를 개발자에게 알려주는 것이다.
B. Replace the second catch block (Fig.11.3) from line 42 to line 47 by the following catch block. Then enter input similar values as shown in the example and execute the program. Explain what result you observed and explain why such type of results occur (including the reason and screen shot of the result , 2 points)
Answer : 'RuntimeException', a more comprehensive exception than 'ArithmeticException', catches the exception that occurs in the try statement.
're' is a variable. 'RuntimeException' before this variable means that the data type of the variable is 'RuntimeException'.
RuntimeException is a class that Java provides by default belongs to java.lang.
When an exception occurs, we are using 're' to create a new runtime exception to invoke the content of the exception.
re는 변수다. 이 변수 앞의 RuntimeException은 변수의 데이터 타입이 RuntimeException이라는 의미다.
RuntimeException은 자바에서 기본적으로 제공하는 클래스로 java.lang에 소속되어 있다.
예외가 발생하면 자바는 마치 메소드를 호출하듯이 catch를 호출하면서 그 인자로 RuntimeException 클래스의 인스턴스를 전달하는 것이다. re를 사용하여 새 런타임 예외를 생성하여 exception의 내용을 호출하고 있다.
e.getMessage()는 자바가 전달한 인스턴스의 메소드 중 getMessage를 호출하는 코드인데, getMessage는 오류의 원인을 사람이 이해하기 쉬운 형태로 리턴하도록 약속되어 있다.
3. After deleting “throws Exception” at line 21(Fig 11.5), compile the program. Explain why a problem happens (including only explanation, 2 points)
Answer : If "throws Exception" is deleted, it cannot re-throw the exception in catch statement of throwException() to the main method which is using the throwException() method
"throws Exception"을 지우면 throwException() 의 catch 문에서 re-throw 하는 exception을 throwException()을 사용하는 main method 에게 넘겨주지 못한다.
// Fig 11.5 : UsingExceptions.java
public class UsingExceptions
{
public static void main(String[] args)
{
try
{
throwException();
}
catch (Exception exception) //throwException에서 에러발생 캐치
{
System.err.println("Exception handled in main");
}
doesNotThrowException();
}
public static void throwException() throws Exception//Exception 에러를 넘긴
{
try
{
System.out.println("Method throwExcpetion"); // (2)
throw new Exception(); // 인위적으로 예외를 발생시킴. 여기서 무조건 Exception이라는 에러 발
} // 이 예외를 처리하기 위해선 throws 로 예외를 밖으로던지거나 try~catch로 처리해줘야 한다.
catch (Exception exception)
{
System.err.println(
"Exception handled in method throwException"); // (1)
}
finally
{
System.err.println("Finally executed in throwException"); // (3)
}
}
public static void doesNotThrowException()
{
try
{
System.out.println("Method doesNotThrowException"); // (5)
}
catch (Exception exception)
{
System.err.println(exception);
}
finally
{
System.err.println(
"Finally excuted in doesNotThrowException"); // (4)
}
System.out.println("End of method doesNotThrowException"); // (6)
}
}
throws Exception이 없을 때 메커니즘
- main함수 안의 try문 안에서 throwException() 실행
- throwException() 안의 try문 실행 : "Method throwException" 출력
- throw new Exception(); // 새로운 Exception을 발생시킨다.
- 바로 throwException() 안의 catch 문에서 새로 발생한 Exception을 잡아주면서
- "Exception handled in method throwException" 출력
- finally 문 실행 : "Finally executed in throwException" 출력
- throwException() 메써드를 실행하고 발생한 예외가 없으므로 main의 catch문을 거치지 않고 doesNotThrowException() 메써드 실행
- doesNotThrowException() 메써드 안에서 try문 실행 : "Method doesNotThrowException" 출력
- doesNotThrwoException() 메써드 안에서 finally 문 실행 : "Finally executed in doesNotThrowException" 출력
- "End of method doesNotThrowException" 출력
throws Exception이 있을 때 메커니즘
- main함수 안의 try문 안의 throwException() 실행
- throwException() 안의 try문 실행 : "Method throwException" 출력
- throw new Exception(); // 새로운 Exception을 발생시킨다.
- 새로 발생한 Exception을 throws 하므로 throwException() 메써드의 사용자인 main이 Exception을 받는게 아니다.
- throwException() 안에서 우선적으로 처리한다. main이 받으려면 catch문 뒤에서 throw exception으로 던져줘야 한다.
- "Exception handled in method throwException" 출력
- finally 문 실행 : "Finally executed in throwException" 출력
- doesNotThrowException() 메써드 실행
- doesNotThrowException() 메써드 안에서 try문 실행 : "Method doesNotThrowException" 출력
- doesNotThrwoException() 메써드 안에서 finally 문 실행 : "Finally executed in doesNotThrowException" 출력
- "End of method doesNotThrowException" 출력
개 헛짓거리 함
main이 받으려면 꼭 catch 문이 뒤에서 throw exception으로 예외를 던져줘야 한다.
// Fig 11.5 : UsingExceptions.java
public class UsingExceptions
{
public static void main(String[] args)
{
try
{
throwException();
}
catch (Exception exception) //throwException에서 에러발생 캐치
{
System.err.println("Exception handled in main");
}
doesNotThrowException();
}
public static void throwException() throws Exception//Exception 에러를 넘긴
{
try
{
System.out.println("Method throwExcpetion"); // (2)
throw new Exception(); // 인위적으로 예외를 발생시킴. 여기서 무조건 Exception이라는 에러 발
} // 이 예외를 처리하기 위해선 throws 로 예외를 밖으로던지거나 try~catch로 처리해줘야 한다.
catch (Exception exception)
{
System.err.println(
"Exception handled in method throwException"); // (1)
throw exception;
}
finally
{
System.err.println("Finally executed in throwException"); // (3)
}
}
public static void doesNotThrowException()
{
try
{
System.out.println("Method doesNotThrowException"); // (5)
}
catch (Exception exception)
{
System.err.println(exception);
}
finally
{
System.err.println(
"Finally excuted in doesNotThrowException"); // (4)
}
System.out.println("End of method doesNotThrowException"); // (6)
}
}
4. After running the following code, what is the output? Why you got this result (including your reason and screen shot of the result, 3 points)
Answer :
(1) Due to the command 'System.out.println ("A");' in the try statement of the main method, "A" is outputted.
(2) When java runs the m() method, due to the command 'System.out.println("E");', "E" is outputted.
(3) RuntimeException is caused by 'throw new RuntimeException();' in if statement.
(4) When the RuntimeException occurs, the catch statement receives Exception e to process the Exception, and "C" is printed.
(5) The finally statement is a logic that is always executed regardless of the occurrence of an exception, so "D" is printed.
- main method의 try문에서 System.out.println("A"); 명령어로 인해 "A"가 출력된다.
- m() method를 실행하면 먼저 System.out.println("E"); 명령어로 인해 "E"가 출력된다.
- if문 안의 throw new RuntimeException(); 명령어로 인해 Exception이 발생한다.
- Exception 발생하면 Exception을 처리하기 위해 catch문이 Exception e 를 받아서 "C"가 출력된다.
- finally 문은 예외가 발생하는 것과 상관없이 언제나 실행되는 logic이므로 "D"가 출력된다.
5. When you compile the following program, an error occurs.
A. Explain why the error happens(1pt)
Answer : This is because after reading a file through FileInputStream(), FileNotFoundException was not made for when the read file did not exist.
FileInputStream을 통해서 파일을 읽은 후, 읽은 파일이 존재하지 않을 때에 대한 예외 처리(FileNotFoundException)가 이루어지지 않았기 때문이다.
B. In order to do normal operation, add the exception handling code using two ways. In your report, include screen shot of errors, explanation for the occurrence of the error, screen shot of the normal code (without error). Include also the source code of the normal code in the source file (6 points)
정상 작동을 위해 두 가지 방법을 사용하여 예외 처리 코드를 추가한다. 보고서에 오류의 스크린샷, 오류 발생에 대한 설명, 일반 코드의 스크린샷(오류 없음)을 포함하십시오. 소스 파일에 일반 코드의 소스 코드도 포함
Screen shot of errors :
explanation for the occurrence of the error : After reading a file through FileInputStream(), FileNotFoundException was not made for when the read file did not exist.
FileInputStream을 통해서 파일을 읽은 후, 읽은 파일이 존재하지 않을 때에 대한 예외 처리(FileNotFoundException)가 이루어지지 않았다.
first normal code(without error) :
second normal code(without error) :
FileInputStream 클래스
자바에서는 파일에서 바이트 단위로 입력할 수 있도록 하기 위해서 FileInputStream 클래스를 제공한다.
이 클래스를 다른 입력 관련 클래스와 연결해서 파일에서 데이터를 읽거나 쓸 수 있다.
FileInputStream 객체를 생성할 때 데이터를 읽어올 파일을 지정한다.
File 객체를 지정하는 방법과 문자열 형태로 파일의 경로명을 지정하는 방법이 있다.
만일 FileInputStream 객체의 생성자에 지정한 파일이 존재하지 않은 경우에는
FileNotFoundException을 발생시킨다.
파일을 생성하는 순서
FileInputStream fis = new FileInputStream("파일 이름");
data = fis.read();
fis.close();
혹은
File f = new File("파일 이름");
FileInputStream fis = new FileInputStream(f);
data = fis.read();
fis.close();
Exception & Error
Error는 실행 중 일어날 수 있는 치명적 오류이다. 컴파일 시점에 체크할 수 없으며 오류가 발생하면 프로그램이 비정상 종료된다.
Exception은 Error보다 비교적 경미한 오류이다. try~catch를 이요해 프로그램의 비정상적인 종료를 막을 수 있다.
Exception
Exception은 크게 Cheched Exception과 Unchecked Exception으로 나뉘는데
Checked Exception은 컴파일 시 체크되는 예외(Exception)로 컴파일 전에 예측이 가능하다.
Unchecked Exception은 컴파일 시 체크되지 않으며 프로그램 실행 도중 발생하는 예외(Exception)로 예측이 불가능하다.
또한 Checked Exception을 발생시킬 수 있는 메서드를 사용할 경우 반드시 throws를 통해 예외를 던지거나 try~catch를 이용해 예외처리해주어야 하는 강제성이 있다.
반면에 Unchecked Exception을 발생시킬 수 있는 메서드를 사용할 경우에는 반드시 예외를 처리해주어야 할 강제성이 없다.
그렇기 때문에 아무래도 Checked 보다는 Unchecked가 개발 편의가 있다고 볼 수 있다.
단, 예외 처리를 하지 않은 상태에서 예외가 발생한다면 오류를 뱉어내며 프로그램이 비정상 종료되므로 주의해야 한다.
위 그림을 보면 알 수 있듯이 RuntimeException은 Unchecked에 속한다.
예외 되던지기 메커니즘 예시로 통달하기 (exception re-throwing)
한 method에서 발생할 수 있는 예외가 여럿인 경우, 몇개는 try-catch로 자체 처리하고
나머지는 선언부에 지정하여 처리하도록 함으로써, 나누어 처리하도록 하는 예외 처리 방법.
예외 되던지기를 모든 함수에 대해 적용하게 되면 프로그램이 돌아가는 flow에 따라 모든 exception을 catch 할 수 있고 (main 에서 최종적으로) 최초 발생 부분에서도 logging 혹은 printing을 통해 exception 발견 종류와 위치를 알 수 있게 된다.
메커니즘
- method1(); // 실행
- throw new Exception(); // 새로운 Exception 만든다.
- catch (Exception e) // 그 새로 만들어진 Exception을 잡는다.
- "method1 메서드에서 예외가 처리되었습니다." // 출력
- throw e; // 예외 새로 다시만든다. method1() 끝난다.
- catch (Exception e) // main으로 돌아와서 method1()에서 마지막에 새로 만들어진 Exception 잡는다.
- "main메서드에서 예외가 처리되었습니다." // 출력
import java.io.IOException;
public class mainClass {
public static void exceptionRethrowing() throws Exception {
try {
System.out.println("4");
throw new IOException("exceptionRethrowing");
} catch (IOException e) {
System.out.println("5");
e.printStackTrace();
throw new Exception("re-throwing");
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
try {
System.out.println("1");
exceptionRethrowing();
System.out.println("2");
} catch (Exception e) {
System.out.println("3");
e.printStackTrace();
System.out.println("end");
}
}
}
메커니즘
- main에서 "1" 출력
- exceptionRethrowing() 메쏘드 실행
- "4" 출력
- throw new IOException("exceptoionRethrowing"); // 새로운 IOException 예외를 만든다.
- catch (IOException e) // 그 예외를 바로 잡는다.
- "5" 출력
- e.printStackTrace(); // 예외 내용 출력한다.
- throw new Exception("re-throwing"); // 새로운 Exception 예외를 만든다.
- exceptionRethrowing() 메쏘드 끝난다. 그리고 throws로 exceptionRethrowing() 메쏘드의 사용자인 main에게 Exception 예외를 던져준다.
- exceptionRethrowing() 메쏘드에서 예외가 발생했으므로 "2"를 출력하지 않고 바로 Exception 예외를 catch로 잡아준다.
예외가 발생하면 그 자리에서 멈춘다. 그리고 바로 catch 문으로 간다. - catch문을 실행하면서 "3"을 출력한다.
- e.printStackTrace(); // 예외 내용 출력한다.
- "end"를 출력하면서 끝낸다.
// A.java
public class A
{
public static void main(String[] args)
{
try
{
System.out.println("A");
m();
System.out.println("B");
}
catch (Exception e)
{
System.out.println("C");
}
finally
{
System.out.println("D");
}
} // end of main() method
public static void m()
{
System.out.println("E");
if (true)
throw new RuntimeException();
System.out.println("F");
}
} // end of class
- main method의 try문에서 System.out.println("A"); 명령어로 인해 "A"가 출력된다.
- m() method를 실행하면 먼저 System.out.println("E"); 명령어로 인해 "E"가 출력된다.
- if문 안의 throw new RuntimeException(); 명령어로 인해 Exception이 발생한다.
- Exception 발생하면 Exception을 처리하기 위해 catch문이 Exception e 를 받아서 "C"가 출력된다.
- finally 문은 예외가 발생하는 것과 상관없이 언제나 실행되는 logic이므로 "D"가 출력된다.
'📌 java > Object-oriented Programming' 카테고리의 다른 글
Homework_12 (0) | 2020.06.02 |
---|---|
Homework_11 (0) | 2020.05.30 |
Homework_9 (0) | 2020.05.13 |
java - use case diagram, scenario (0) | 2020.05.09 |
ATM_Implementation (0) | 2020.05.09 |