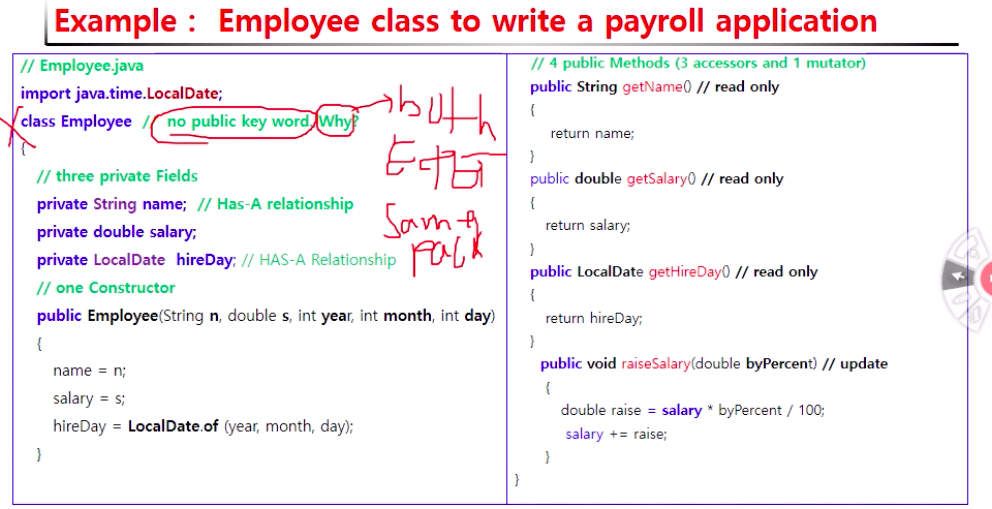
// Employee.java
import java.time.LocalDate;
//no public key word. Why?
class Employee
{
// three private Fields
private String name; // Has-A relationship
private double salary;
private LocalDate hireDay; // HAS-A Relationship
// one Constructor
public Employee(String n, double s, int year, int month, int day)
{
name = n;
salary = s;
hireDay = LocalDate.of (year, month, day);
}
// 4 public Methods (3 accessors and 1 mutator)
public String getName() // read only
{
return name;
}
public double getSalary() // read only
{
return salary;
}
public LocalDate getHireDay() // read only
{
return hireDay;
}
public void raiseSalary(double byPercent) // update
{
double raise = salary * byPercent / 100;
salary += raise;
}
}
// EmployeeTest.java
// import Employee; // error why?
// save as EmployeeTest.java
public class EmployeeTest
{
public static void main(String[] args)
{
// fill the staff array with three Employee objects
Employee[] staff = new Employee[3];
staff[0] =
new Employee("Carl Cracker", 75000, 1987, 12, 15);
staff[1] =
new Employee("Harry Hacker", 50000, 1989, 10, 1);
staff[2] =
new Employee("Tony Tester", 40000, 1990, 3, 15);
// raise everyone's salary by 5%
for (Employee e : staff)
e.raiseSalary(5);
// print out information about all Employee objects
for (Employee e : staff)
System.out.println("name=" + e.getName() + ",salary=" + e.getSalary() + ", hireDay=" + e.getHireDay());
}
}
왜 public 이 필요 없냐 Employee.java 에?
Employee.java와 EmployeTest.java 둘다 같은 default package 에 속하기 때문이다.
Note 3
: main이 EmployeeTest.class에 있기 때문이다.
'📌 java > Object-oriented Programming' 카테고리의 다른 글
java - public의 의미 (0) | 2020.03.27 |
---|---|
Homework_W2-02 (0) | 2020.03.27 |
LotteryDrawing.java (0) | 2020.03.26 |
java - txt.file (0) | 2020.03.26 |
java.util.Arrays - Arrays 클래스 (0) | 2020.03.26 |