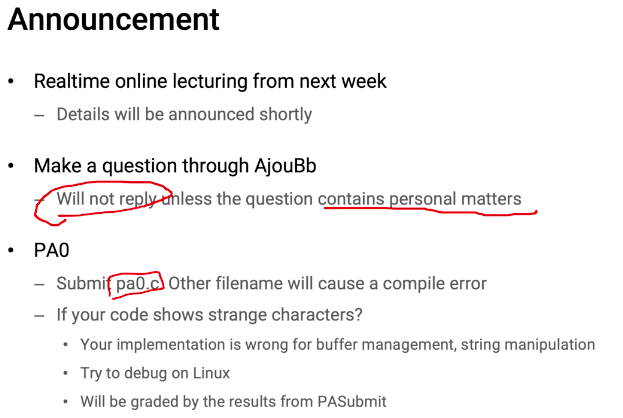
파일 이름 pa0.c로 해라
리눅스에서 되는거만 인정
pdf 한장으로 제출. 이름 학번 넣을 필요 X
ICT332 Operating Systems (Spring 2020)
Project #0: Implementing a Command Line Parser
Due on the end of March 29 (Sunday) (23:59:59)
Introduction
The purpose of this project is to bootstrap the term project to implement a shell program, and to get familiar with the programming assignment submission system.
이 프로젝트의 목적은 셸 프로그램을 구현하기 위한 용어 프로젝트와 프로그래밍 할당 제출 시스템에 익숙해지는 것이다.
Problem Specification
We will implement a shell program soon. To that end, you should firstly make the shell program to understand user inputs and commands.
곧 셸 프로그램을 시행할 것이다. 그러기 위해서는 먼저 셸 프로그램을 만들어 사용자 입력과 명령을 이해해야 한다.
In this project, the framework passes input strings, and your task is to split the input strings into command tokens.
이 프로젝트에서 프레임워크는 입력 문자열을 pass하며, 과제는 입력 문자열을 command 토큰으로 나누는 것이다.
By C standard, a string is defined as a sequence of characters which is terminated with \0.
C standard에 따르면 문자열은 \0으로 끝나는 문자열로 정의된다.
Command tokens are strings that are obtained by splitting the input string with delimitors which is whitespace characters in this PA.
command 토큰은 입력 문자열을 이 PA의 공백 문자인 구분 기호로 분할하여 얻은 문자열이다.
Use isspace()function to check whether a character is whitespace or not.
문자의 공백 여부를 확인하려면 isspace() 기능을 사용하라.
Any heading and trailing whitespace should be removed from the command tokens.
명령 토큰에서 heading 및 trailing 공백은 제거해야 한다. 처음과 끝 공백
For example, if the input string is " Hello world ", the command tokens are "Hello" and "world".
예를 들어 입력 문자열이 " Hello world "인 경우, command 토큰은 "Hello"와 "world"이다.
Note that the input string is split into tokens delimited by whitespaces (' ' in this example), and the tokens do not contain whitespaces.
입력 문자열은 백스페이스(이 예에서는 ' ' ')로 구분된 토큰으로 분할되며 토큰에는 백스페이스가 포함되지 않는다는 점에 유의하십시오.
Another example string is " cp a.out pa0.c /tmp ". It should be broken into "cp", "a.out", "pa0.c", and "/tmp".
또 다른 예제 문자열은 "cp a.out pa0.c /tmp "이다. "cp", "a.out", "pa0.c", "/tmp"로 구분해야 한다.
Skeleton Code
The required functionality must be implemented in parse_command() function in pa0.c. The user input is passed through command.
필요한 기능은 반드시 pa0.c의 parse_command() 함수로 구현해야 한다. 사용자 입력은 command를 통해 전달된다.
After parsing command into tokens, the tokens should be assigned to tokens[] in order, which is passed as the argument of the parse_command() function.
명령을 토큰으로 구문 분석한 후, 토큰을 순서대로 토큰[]에 할당해야 하며, 이는 parse_command() 함수의 인수로 전달된다.
Also, the number of tokens should be assigined to *nr_tokens which will be used by the framework.
또한 토큰의 수는 프레임워크에서 사용할 *nr_tokens로 한정되어야 한다.
Below diagram illustrates a correct result for an input string " ls -al /home/ict332 ".
Note that each token is also a string which is terminated with \0.
아래 다이어그램은 입력 문자열 "ls -al /home/ict32 "에 대한 올바른 결과를 보여준다.
각 토큰도 \0으로 끝나는 문자열이라는 점에 유의하십시오.
char *command --> " ls -al /home/ict332 "
*nr_tokens = 3
tokens[0] --> "ls"
tokens[1] --> "-al"
tokens[2] --> "/home/ict332"
tokens[3]... = NULL
Restrictions
- You should not use any string manipulation functions from any libraries. The banned functions include strtok, strtok_r, strlen, fscanf and/or similars. This implies that you should implement your own string manipulation functions if it is needed. You will get 0 point if you use any of them. Note that malloc() and free() are not string manipulation functions and OK to use. If unsure, question to TA/professor through AjouBb.
- Use isspace() C library function to check whether the character can be assumed as a whitespace.
- Printing messages to stand output (e.g., printf()) is totally OK. However, do not fprintf(stderr ...) otherwise the grading system cannot grade your submission properly.
Logistics
-
This is an individual project; you work on the assignement alone.
-
You can use up to 4 slip tokens throughout this semester.
-
You can start this program assignment by cloning this repository at https://github.com/sslab-ajou/ict332-pa0-2020s. Everything is in pa0.c. Please do not modify the main() function, but implement your code in parse_command(). You can add any functions or macros if you need, for sure.
-
This is an OS/architecture-independent project; you may use Visual Studio on Windows or vim/gcc on Linux. However, the grading will be done on a Linux machine. So, write code as neutral as possible to compilers.
-
All submission and grading are done automatically through https://sslab.ajou.ac.kr/pasubmit. Please follow the instruction explained in the class.
- Submit pa0.c file to the PA submission site. (80 pts)
- Explain your parsing strategy on a 1-page PDF document. Submit the document as the report in the PASubmit. (20 pts)
-
input file in the provided code contains the input sequence for the grading.
Tips and Notes
- Briefly speaking, the implementation might look like; start scanning from the beginning of command skipping all whitespaces until meet a non-whitespace character. That will be the beginning of the token. Then, resume scanning until meet any whitespace character (' ' or '\t'). That will be the end of the token. Repeat this until entire commandis scanned.
- Read comments in the skeleton code carefully.
- Make sure every string is terminated with '\0'.
- Post freely on QnA board on AjouBb to question about the project.
Good luck and have fun!
/**********************************************************************
* Copyright (c) 2020
* Sang-Hoon Kim <sanghoonkim@ajou.ac.kr>
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 2 as
* published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTIABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
**********************************************************************/
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <ctype.h>
/* Suppress security errors on Visual Studio */
#define _CRT_SECURE_NO_WARNINGS
#pragma warning(disable : 4996)
#define MAX_NR_TOKENS 32 /* Maximum number of tokens in a command */
#define MAX_TOKEN_LEN 64 /* Maximum length of single token */
#define MAX_COMMAND 256 /* Maximum length of command string */
typedef unsigned char bool;
#define true 1
#define false 0
/***********************************************************************
* parse_command
*
* DESCRIPTION
* Parse @command, put each command token into @tokens[], and
* set @nr_tokens with the number of tokens.
*
* A command token is defined as a string without any whitespace (i.e., *space*
* and *tab* in this programming assignment). Suppose @command as follow;
*
* @command = " Hello world Ajou University!! "
*
* Then, @nr_tokens = 4 and @tokens should be
*
* tokens[0] = "Hello"
* tokens[1] = "world"
* tokens[2] = "Ajou"
* tokens[3] = "University!!"
*
* Another exmaple is;
* command = "ls -al /home/operating_system /hw0 "
*
* then, nr_tokens = 4, and tokens is
* tokens[0] = "ls"
* tokens[1] = "-al"
* tokens[2] = "/home/operating_system"
* tokens[3] = "/hw0"
*
*
* RETURN VALUE
* Return 0 after filling in @nr_tokens and @tokens[] properly
*
*/
static int parse_command(char *command, int *nr_tokens, char *tokens[])
{
/**
* TODO
*
* Followings are some example code. Delete them and implement your own
* code here
*/
tokens[0] = "hello";
tokens[1] = "world";
*nr_tokens = 2;
return 0;
}
/***********************************************************************
* The main function of this program.
* SHOULD NOT CHANGE THE CODE BELOW THIS LINE
*/
int main(int argc, char *argv[])
{
char line[MAX_COMMAND] = { '\0' };
FILE *input = stdin; //
if (argc == 2) {
input = fopen(argv[1], "r");
if (!input) {
fprintf(stderr, "No input file %s\n", argv[1]);
return -EINVAL;
}
}
while (fgets(line, sizeof(line), input)) {
char *tokens[MAX_NR_TOKENS] = { NULL };
int nr_tokens= 0;
parse_command(line, &nr_tokens, tokens);
fprintf(stderr, "nr_tokens = %d\n", nr_tokens);
for (int i = 0; i < nr_tokens; i++) {
fprintf(stderr, "tokens[%d] = %s\n", i, tokens[i]);
}
printf("\n");
}
if (input != stdin) fclose(input);
return 0;
}
'🚦 Server > Operating System' 카테고리의 다른 글
04. Operating System Structures (2) (0) | 2020.03.30 |
---|---|
03. Operating System Structures (1) (0) | 2020.03.25 |
02. System Call (0) | 2020.03.23 |
01. Introduction to OS (0) | 2020.03.18 |
00. Welcome aboard ICT332 (0) | 2020.03.16 |